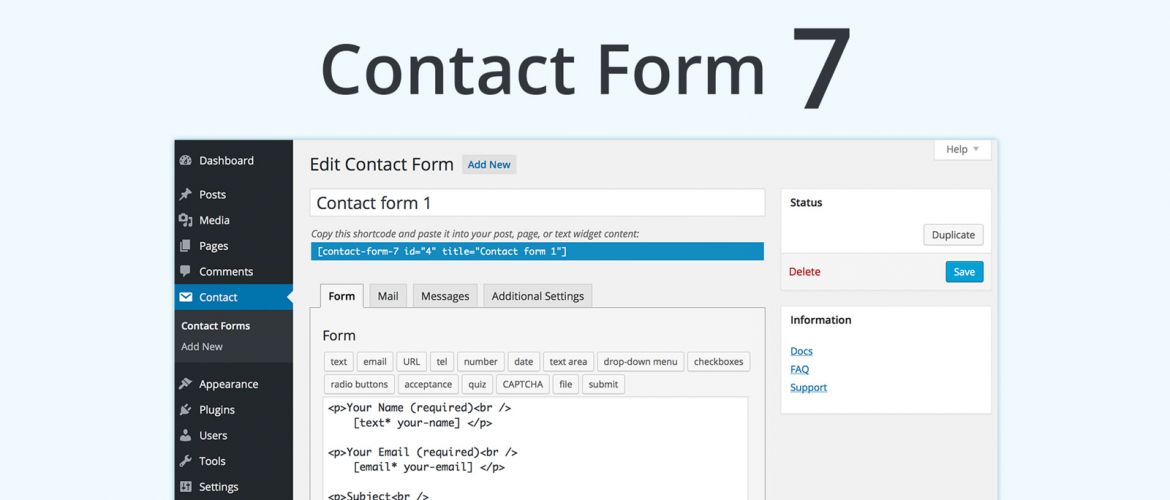
Here as we already know that WordPress Plugin Contact Form 7 is very popular for WordPress forms. So, sometimes we also need Contact Form 7 custom validation for some fields like Full Name, Email Address, Phone Number, etc. In WordPress, Contact Form 7 Validation is very easy as I have given below:
Contact Form 7 provides several kinds of user-input validation by default, including:
- Is a required field filled in?
- Does an email field have an email address in the correct format?
- Is an uploaded file in an acceptable file type and size?
But You can also add your own custom validation.
Contact Form 7 have 2 ways of Validations:
1. WordPress Filters( Validation as a filter ):
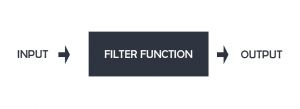
You can use WordPress filters for Contact Form 7. Form adding a filter, you need to custom validation function/filter in function.php file.
In Contact Form 7, a user-input validation is implemented as a filter function. The filter hook used for the validation varies depending on the type of form-tag and is determined as: wpcf7_validate_
+ {type of the form-tag}. So, for text form-tags, the filter hook is used. Likewise, wpcf7_validate_email*
is used for email* form-tags.
Let’s say you have the following email fields in a form:
Email: [email* your-email] Name: [text* your-name] Job Tititle: [text* your-job]
The following listing shows code that verifies whether the two fields have identical values.
//Name Validaiton for Job Titile or Full Name
add_filter( 'wpcf7_validate_text*', 'custom_text_validation_filter', 50, 2 );
function custom_text_validation_filter( $result, $tag ) {
if ( 'your-name' == $tag->name ) {
$your_name = isset( $_POST['your-name'] ) ? trim( $_POST['your-name'] ) : '';
if (preg_match('/[0-9]/', $your_name)) {
$result->invalidate( $tag, "The field contains numeric." );
}
}
if ( 'your-job' == $tag->name ) {
$your_job = isset( $_POST['your-job'] ) ? trim( $_POST['your-job'] ) : '';
if (preg_match('/[0-9]/', $your_job)) {
$result->invalidate( $tag, "The field contains numeric." );
}
}
return $result;
}
//Email Validation already exits or not
add_filter( 'wpcf7_validate_email*', 'custom_email_validation_filter', 50, 2 );
function custom_email_validation_filter( $result, $tag ) {
if ( 'your-email' == $tag->name ) {
$your_email = isset( $_POST['your-email'] ) ? trim( $_POST['your-email'] ) : '';
global $wpdb;
$cf7table = $wpdb->prefix.'db7_forms';
$db = $wpdb->get_results ( "SELECT * FROM $cf7table WHERE `form_value` LIKE '%".$your_email."%' ");
//echo $wpdb->num_rows;
//print_r($db);
if ( $wpdb->num_rows > 0) {
$result->invalidate( $tag, "The email is already exist." );
}
}
return $result;
}
2. Jquery Validation Plugin For Contact Form 7
This plugin adds jquery form validation to your contact form 7. You will be able to validate extra validation rules like URL, date, credit card, phone number in the contact form 7 fields.
Benefits you get from these plugins.
- Adds Jquery Validation to Contact Form Fields.
- Faster validation than of contact form core validation.
- Allows the field to be validated for URL, date, credit card, number, and more.
- Field Highlight features for invalid field data types.
- Error message for individual fields.
- Easy to add validation rules. Just add the class in contact form 7 fields.
- Interactive validation that your users love.
- Works with multiple forms now.
Validation Methods are available in the lite version of Contact form 7.
- Required
- Url
- Date
- Number
- Digit only
- Credit Card
- US Phone number
- Letters Only
- IBAN (International Bank account number)
Download Jquery Validation Plugin
Additional Validation Methods are available in the PRO version.
- Multiple Custom Code Validation with One time Code option.
- Custom RegEx Validation – Define any validation rules you need.
- Username validation check (Demo : https://bit.ly/2BueOn2).
- Email Code Verification (Demo: https://bit.ly/3gOJuiS).
- Alpha Numeric – Letters, numbers, and underscores only
- Bank or Giro account number.
- BIC Code – Accepts Valid BIC Code Only.
- Giro Account – Accepts Giro Account number only.
- Integer – Accepts positive or negative non-decimal number.
- IPv4 / IPv6 – Accepts IPv4/IPv6 IP address only.
- Mobile UK – Accepts UK Mobile number only.
- Phone UK – Accepts UK phone number only.
- US State – Accepts US state only.
- Minimum Number – Makes the element require a given minimum.
- Maximum Number – Makes the element require a given maximum.
- Minimum Length – Makes the element require a given minimum length.
- Maximum Length – Makes the element require a given maximum length.
- Range Length – Makes the element require a given value range.
- Range – Makes the element require a given value range.
- Equal To – Requires the element to be the same as another one
- Extension – Makes the element require a certain file extension
- Require Group – Ensures a given number of fields in a group are complete.
Summary for Custom Validation in Contact Form 7 for Phone Number, Email, Name in WordPress.
Basically, the First Method ( WordPress Filters) is a Free and better way to add custom or extra validation in Contact Form 7. Because WordPress Filters gives you free hand for any kind of validation in form and it’s free to use. But the Plugin has limited features in the free version. So for full features, you need to buy the PRO version.
- Install and activate the “Contact Form 7” plugin on your WordPress site.
- Create a new form or edit an existing one in Contact Form 7.
- Add the name and email fields to your form using the following code:
[text* your-name]
[email* your-email]
- Add a custom validation function for the name and email fields using the following code:
function custom_email_validation_filter($result, $email) { $your_name = $_POST['your-name']; $your_email = $_POST['your-email']; if(empty($your_name) || empty($your_email)) { $result->invalidate($email, "Please enter a valid name and email address."); } return $result; } add_filter('wpcf7_validate_email*', 'custom_email_validation_filter', 10, 2);
- Save your changes and preview your form to ensure that the custom validation is working properly.
With these steps, you have successfully implemented custom validation for name and email fields in Contact Form 7. This will help prevent spam and ensure that only valid data is submitted through your forms. You can modify the validation function to include additional validation rules if necessary.
Also Read, Top 10 Programming Languages to start and Best Language for Freelancing
Contact form 7 validation before submit. Is it Possible?
Yes, it is possible to validate a Contact Form 7 form before it is submitted using JavaScript. Here’s how you can do it:
- Add an ID to your form tag in Contact Form 7. For example:
<form id="my-contact-form" method="post" action="your-action-url">
- Create a new JavaScript file or add the following code to your existing JavaScript file:
document.addEventListener( 'wpcf7submit', function( event ) {
var form = document.querySelector('#my-contact-form');
if (form.checkValidity() === false) {
event.preventDefault();
// Show an error message or alert
alert('Please fill in all required fields before submitting the form.');
}
}, false );
- Include the JavaScript file on the page where your Contact Form 7 form is displayed. Make sure to load the JavaScript file after the Contact Form 7 script has loaded.
With this code, the form will be validated before it is submitted, and an error message will be displayed if any required fields are left empty. You can modify the error message or the validation rules to suit your needs.