Basically, variables are used to stored data used and used again and again. here in PHP 7 variable similarly works and there are many kinds of variable declaration
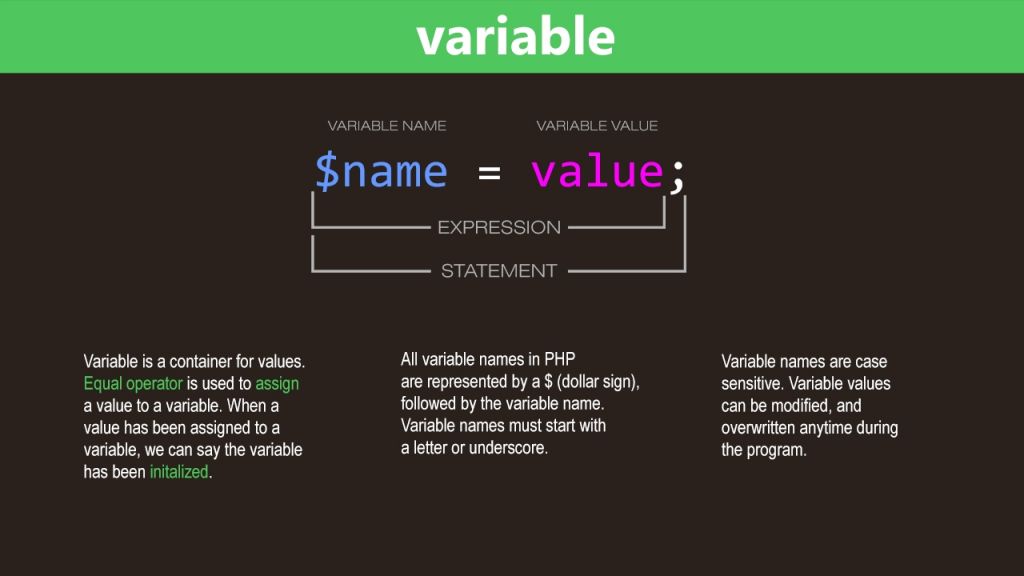
PHP 7 variable are 4 types:
- PHP Local variables
- PHP Global variables
- PHP Super Global variables
- PHP Static variables
Basically, There are 3 types of Local, Global, and Static variables in PHP 7.
- String variables
- Numeric variables
- Boolean variables
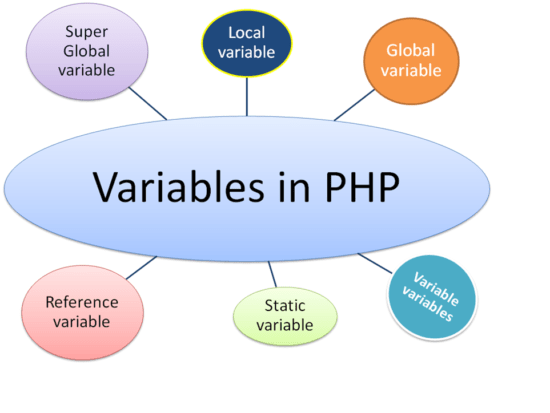
1. PHP Local variables – How to Define and use PHP 7 Local variables.
PHP Local variables have some restrictions as well as limitations. As we have shown in the Example below. Basically, PHP Local variables have scope only inside the class, methods( function).
<?php //here we define a function which return value of variable $name. function test1() { $name ='smith'; // local variable return $name; } echo test1(); // calling a function to get variable value. echo $name; // give a undefine error ?>
Output:
smith Undefined variable: name in C:\wamp64\www\practice php\DEMO.PHP on line 10
2. PHP Global variables – Define and Use of PHP 7 Global variables.
Basically, PHP Global variables have no restrictions. As we have shown in the Example below. PHP Global variables have a scope on all over the page. means you can be used variable wherein a single page
<?php $name ='smith';// define simple variable //here we define a function which return value of variable $name. function test1() { global $name; // global keyword makes a variable global an used inside a function return $name; } echo test1()."<br>"; // calling a function to get variable value. echo $name; // give a value 'smith' ?>
Output:
smith smith
3. PHP Super Global variables
Basically, PHP Super Global variables are totally different from other categories of variables. So, the word carries data from one page to another. Let shown in the example given below.
$_POST,$_GET and $_SESSION etc are superglobal variables read in Upcoming tutorials
<?php $_POST[name];// define super global variable ?>
4. PHP Static variables
Basically, Static variables in PHP are a little bit different from others. they are stored value on every calling them. let see in the example.
<?php function test1() { static $name =0; // global keyword makes a variable global an used inside a function $name++; // adding 1 to $name return $name; } echo test1()."<br>"; // calling a function to get variable value. echo test1()."<br>"; // calling a function again to get variable value. echo test1(); // calling a function again to get variable value. ?>
Output:
0 1 2
when we call first time function test1() it returns 1 [0 + 1] and on calling second time it returns 2[ 1 + 1]. Because static variable declares only one time than it stores last value and added to new.
Now, How to declare PHP 7 variables
1. PHP String variables
Imagine you have a matchbox on which you have written the word username. You then write Fred Smith on a piece of paper and place it into the box. Well, that’s the same process as assigning a string value to a variable, like this:
$username = "Fred Smith";
The quotation marks indicate that “Fred Smith” is a string of characters. You must enclose each string in either quotation marks or apostrophes (single quotes), although there is a subtle difference between the two types of the quote, which is explained later. When you want to see what’s in the box, you open it, take the piece of paper out, and read it. In PHP, doing so looks like this:
echo $username;
Or you can assign it to another variable (photocopy the paper and place the copy in another matchbox), like this:
$current_user = $username;
If you are keen to start trying out PHP for yourself, you could try entering the examples in this chapter into an IDE (as recommended at the end of Chapter 2) to see instant results or you could enter the code in Example 3-4 into a program editor and save it to your server’s document root directory (also discussed in Chapter 2) as test1.php.
Example 3-4. Your first PHP variable declaration program
<?php // test1.php ( Save Page as test1.php in local disk C: > Wamp >www > test1.php) $username = "Fred Smith"; echo $username; echo "<br>"; $current_user = $username; echo $current_user; ?>
Now you can call it up by entering the following into your browser’s address bar:
http://localhost/test1.php
The result of running this code should be two occurrences of the name “Fred Smith,”
the first of which is the result of the echo $username command, and the second of the
echo $current_user command.
If during installation of your web server (as detailed in Chapter 2)
you changed the port assigned to the server to anything other than
80, then you must place that port number within the URL in this and
all other examples in this book. So, for example, if you changed the
port to 8080, the preceding URL becomes:
http://localhost:8080/test1.php
I won’t mention this again, so just remember to use the port num‐
ber if required when trying out any examples or writing your own
code
PHP Numeric variables
Variables don’t contain just strings—they can contain numbers, too. If we return to the
matchbox analogy, to store the number 17 in the variable $count, the equivalent would
be placing, say, 17 beads in a matchbox on which you have written the word count:
$count = 17;
You could also use a floating-point number (containing a decimal point); the syntax is
the same:
$count = 17.5;
To read the contents of the matchbox, you would simply open it and count the beads.
In PHP, you would assign the value of $count to another variable or perhaps just echo
it to the web browser.
PHP 7 Variable naming rules – Super Global, String, numeric and all other Variables
When creating PHP variables, you must follow these four rules:
• Variable names must start with a letter of the alphabet or the _ (underscore)
character.
• Variable names can contain only the characters a–z, A–Z, 0–9, and _ (underscore).
• Variable names may not contain spaces. If a variable must comprise more than one
word, it should be separated with the _ (underscore) character (e.g., $user_name).
• Variable names are case-sensitive. The variable $High_Score is not the same as the
variable $high_score.
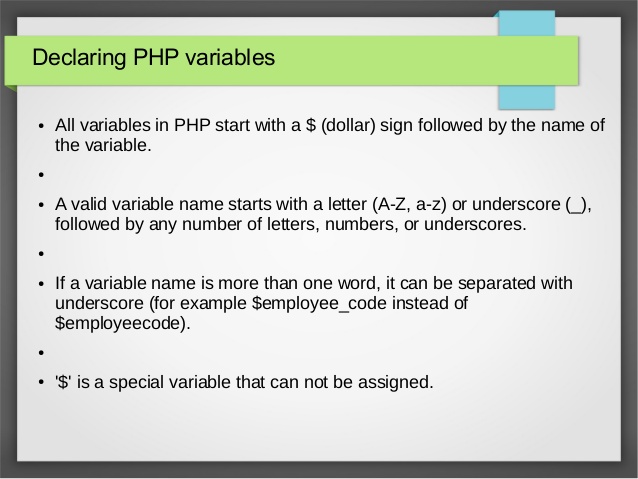
To allow extended ASCII characters that include accents, PHP also supports the bytes from 127 through 255 in variable names. But un‐
less your code will be maintained only by programmers who are
familiar with those characters, it’s probably best to avoid them, be‐
cause programmers using English keyboards will have difficulty ac‐
cessing them.
The basic FAQ of PHP 7 Variables
A global variable can be declared just like other variable but it must be declared outside of function definition. Global variables refer to any variable that is defined outside of the function. Global variables can be accessed from any part of the script i.e. inside and outside of the function.
Global variables are stored in the Data Segment of the process. Global variable’s life is until the life of the program and it can be accessed by other files using extern keyword.
A static variable can be declared outside of all functions or within a function using a static keyword before the data type of variable